There are many times a Magento 2 controller should be forwarded to a 404 (noroute
) path – making a GET
request for a path that should only respond to a POST
request, a dynamic controller that allows some but not all paths, etc. This sounds simple, right? It is, but for some reason I always forget the proper syntax and spend way too long looking it up… Hopefully this post can save you some time like I feel it will for me.
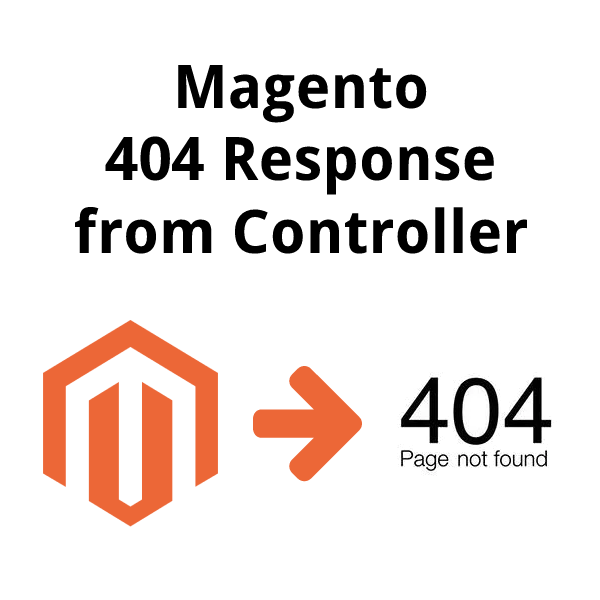
The Magento 2 router can be used to forward any request to another action/controller as needed. In the case of this tutorial, the request needs to be forwarded to what Magento calls the noroute
action. The noroute
action will set the header Status Code
to 404
(not found). The configured Magento 404 page will be returned to the visitor.
Use Magento ResultFactory
to Redirect to noroute
The Magento framework class of Magento\Framework\Controller\ResultFactory
[source] is used to forward to the noroute
action. This handles setting the proper headers and obtaining the proper 404
page content from Magento.
1 2 3 4 | /** @var Forward $resultForward */ $resultForward = $this->resultFactory->create(ResultFactory::TYPE_FORWARD); $resultForward->forward('noroute'); return $resultForward; |
Controller Example
Here’s a very simplified example controller that checks if the request has POST
data. If it does, process the controller logic. If not, return a 404
(or noroute
as Magento calls it) response.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 | # file: app/code/Vendor/Namespace/Controller/ActionName.php <?php namespace Vendor\Namespace\Controller; use Magento\Framework\Controller\ResultFactory; class ActionName { /** * @var resultFactory */ protected $resultFactory; /** * @param ResultFactory $resultFactory */ public function __construct( ResultFactory $resultFactory ) { $this->resultFactory = $resultFactory; } public function execute() { $postData = $this->getRequest()->getPostValue(); if( empty( $postData ) ) { // no post data, thus return a 404 /** @var Forward $resultForward */ $resultForward = $this->resultFactory->create(ResultFactory::TYPE_FORWARD); $resultForward->forward('noroute'); return $resultForward; } else { // valid request, process controller logic } } } |